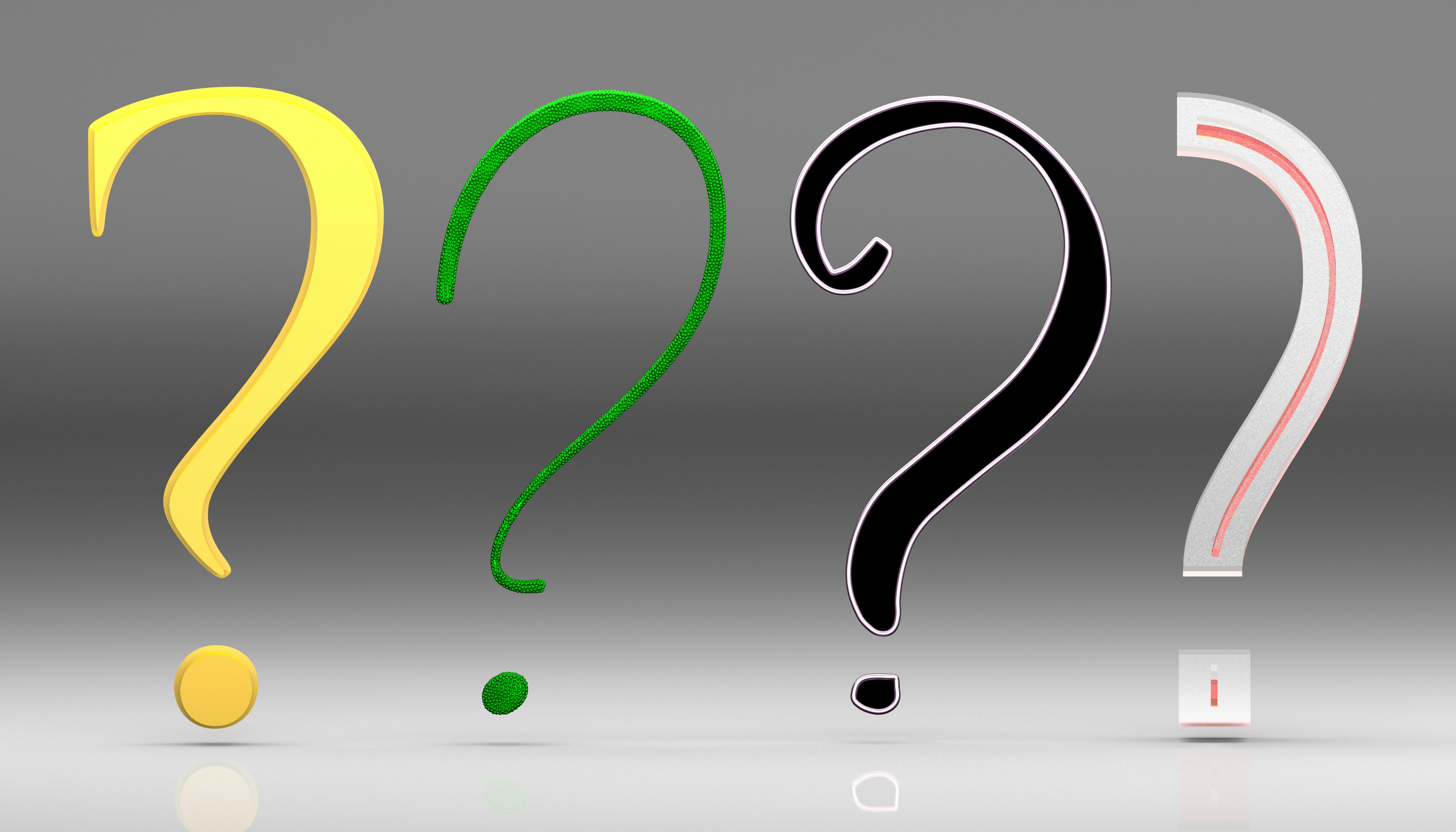
Data Engineering Job Interview Warm‑Up: 30 Real Coding & System‑Design Questions
The world of data engineering has rapidly emerged as a critical pillar for businesses, enabling them to extract insights from vast amounts of information and power data-driven decision-making. From building scalable ETL pipelines to designing real-time streaming infrastructures and cloud data warehouses, data engineers are in high demand across every industry—from tech giants to healthcare providers to financial institutions.
If you’re seeking a data engineering role, you may already know that interviews can be rigorous, spanning software development, database design, distributed systems, and cloud computing. Many organisations need engineers who can handle both traditional batch processing and cutting-edge real-time analytics frameworks, all while keeping data secure, consistent, and optimised.
In this guide, we’ll explore 30 real coding & system-design questions that often come up in data engineering interviews. From classic coding challenges to architecture-focused scenarios, these questions will help you gauge your readiness and build confidence before stepping into that interview room.
If you’re actively searching for new data engineering opportunities in the UK, www.dataengineeringjobs.co.uk is a fantastic resource. It features a wide range of vacancies—from junior data engineering positions to senior-level cloud architecture roles. Let’s dive in so you can approach your next interview with insight and poise.
1. Why Data Engineering Interview Preparation Matters
Data engineering has evolved into a multidisciplinary field combining elements of software development, database systems, big data processing frameworks, and cloud-native design. Employers look for candidates who can ensure data pipelines are robust, scalable, and secure. Here’s why structured interview prep is crucial:
Showcase Technical Breadth
You may be asked to design entire end-to-end data architectures, from ingestion to consumption.
Demonstrate strong command over SQL, NoSQL, Python, Scala, Java, or other relevant languages.
Emphasise System Design & Scalability
Data engineering solutions typically handle massive datasets.
Interviews often require you to discuss distributed systems (Hadoop, Spark, Kafka) and strategies for partitioning, sharding, or replication.
Highlight Practical, Production-Ready Solutions
It’s not just about theory; recruiters want to see if you can implement data pipelines in real-world settings, addressing monitoring, logging, retries, and error handling.
Expect to discuss DevOps or DataOps principles like CI/CD for data pipelines.
Validate Communication & Problem-Solving
Data engineers often collaborate with data scientists, analysts, and business stakeholders.
Clear communication of complex data flows and trade-offs is as important as coding prowess.
Demonstrate Knowledge of Cloud Platforms
With more organisations migrating to the cloud, familiarity with AWS, Azure, or GCP (and their managed data services) is hugely beneficial.
You might be asked how you’d leverage S3, EMR, Redshift, BigQuery, Synapse, or similar products.
By tackling both coding and system design questions, you’ll be equipped to show interviewers you can build, automate, and optimise sophisticated data solutions. Let’s begin with 15 coding challenges frequently posed in data engineering interviews.
2. 15 Real Coding Interview Questions
While data engineering revolves around architecture and large-scale processing, coding tests remain a core part of the interview. Below are 15 coding prompts that often appear, touching on data manipulation, algorithms, and platform-specific use cases.
Coding Question 1: ETL Parsing & Validation
Question: You receive a CSV file daily with user data. Write code to parse each record, validate mandatory fields (like user_id), and store valid rows in a data structure for further processing.
What to focus on:
Efficient file I/O and error handling.
Data structure selection (list, dict, or custom class).
Logging invalid rows for auditing.
Coding Question 2: Data Deduplication
Question: Implement a function to remove duplicate records from a list of user objects, retaining only the latest based on a timestamp field.
What to focus on:
Hashing or dictionary-based solutions to detect duplicates.
Comparing timestamps to decide which record to keep.
O(n) or O(n log n) approaches, depending on data size.
Coding Question 3: Merge Sort with Memory Constraints
Question: Merge two large sorted datasets that each exceed memory capacity. Show how you’d do this in a streaming/batch manner.
What to focus on:
External merge sort principles.
Chunking data to avoid memory overflow.
Handling I/O efficiently (buffered reads/writes).
Coding Question 4: String Tokenisation for Data Pipelines
Question: Write a function to split large text fields into tokens, removing stop words and normalising case. Return a list of unique tokens for each row.
What to focus on:
String processing (regex or built-in split methods).
Data normalisation (case folding, punctuation removal).
Token indexing or frequency mapping if required.
Coding Question 5: Real-Time Data Filtering
Question: Given a continuous stream of JSON events (e.g., from Kafka), implement a function that filters out events missing critical fields (e.g., event_type).
What to focus on:
Reading from a streaming source in a language of choice (Python, Java, Scala).
JSON parsing libraries and error handling.
Efficient checks with minimal latency overhead.
Coding Question 6: Sorting and Aggregation
Question: You have sales records (order_id, date, amount). Implement logic to group by date and sum total amounts for each date, returning a dictionary keyed by date.
What to focus on:
Basic grouping patterns (hash map, combiners).
Summation logic that accommodates big integer sums if needed.
Handling edge cases like missing dates or partial records.
Coding Question 7: Implement a Window Function
Question: Code a custom sliding window function to compute moving averages over a list of numerical values, given a window size.
What to focus on:
Data structure to maintain the current window (queue or deque).
Time complexity (adding/removing elements from the window).
Handling window edges (initial fill, trailing windows).
Coding Question 8: Parallelising a MapReduce Task
Question: Demonstrate how you’d implement a simple word count using a map-and-reduce approach. Assume you can split the data across multiple workers.
What to focus on:
Map tasks for tokenisation.
Shuffle and sort or direct partitioning of keys.
Reduce tasks for summing counts.
Coding Question 9: SCD (Slowly Changing Dimension) Handling
Question: Write a function to merge incoming dimension table updates (e.g., user address changes) into an existing table. Manage old records via start_date and end_date.
What to focus on:
SCD Type 2 approach (new row insertion, old row closure).
Checking if changes exist or if fields remain unchanged.
Correct date/time fields to track historical data.
Coding Question 10: Binary File Serialisation
Question: Serialise a list of custom objects (e.g., user profiles) into a binary file format and then read them back, preserving all fields accurately.
What to focus on:
Data schema consistency.
Handling variable-length strings or nested objects.
Error handling for corrupted data during deserialisation.
Coding Question 11: Regex-Based Validation
Question: Implement a function that checks if a string follows a pattern, e.g., an email address or IP address, using regular expressions.
What to focus on:
Efficient regex compilation or usage.
Edge cases (empty string, invalid domain segments, etc.).
Potential performance issues with complex patterns.
Coding Question 12: Graph Data Processing
Question: You have edges describing relationships between users. Implement a function to find connected components in this graph.
What to focus on:
Graph traversal (DFS/BFS).
Data structure for adjacency lists or sets.
Handling large graphs with memory constraints.
Coding Question 13: Handling Out-of-Order Events
Question: In a streaming data pipeline, events can arrive late. Show how you’d reorder events (by timestamp) before processing, given a defined “lateness threshold.”
What to focus on:
Buffering events until they can be sorted by timestamp.
Dropping or marking very late events.
Balancing memory usage and latency.
Coding Question 14: RESTful API Data Ingestion
Question: Write a Python function that fetches data from a REST API, handles pagination, and stores responses in a local database.
What to focus on:
API pagination logic (next page tokens, offsets).
Rate limit handling (retry with backoff).
Inserting data efficiently (batch commits).
Coding Question 15: Encryption in Transit
Question: Implement a function that sends data securely to a remote server using TLS. Show how you’d verify the server’s certificate.
What to focus on:
Setting up secure connections (SSL/TLS libraries).
Handling certificate verification or pinning.
Mitigating man-in-the-middle attacks.
In your coding solutions, aim for clarity, modularity, and efficiency. Where relevant, consider scalability and fault tolerance, reflecting real data engineering pipeline needs.
3. 15 Data Infrastructure & Architecture Questions
Beyond coding, data engineering interviews often involve system or architecture design sessions. Here, you’ll be asked how you’d build and operate scalable, robust data systems—ranging from on-premises clusters to cloud-native stacks. Below are 15 key architecture questions that probe your knowledge of big data frameworks, data warehousing, streaming, and more.
Architecture Question 1: Batch Data Pipeline on Hadoop
Scenario: You have daily log files, each up to several gigabytes, and you need to run transformations to create aggregated reports.
Key Points to Discuss:
Job orchestration (e.g., Apache Airflow, Oozie).
Storage (HDFS, S3) and compute (Hadoop MapReduce, Spark).
Partitioning and scheduling for efficiency.
Architecture Question 2: Real-Time Analytics with Kafka & Spark
Scenario: You must process clickstream events in near real time, updating dashboards for user activity.
Key Points to Discuss:
Apache Kafka for ingestion and buffering.
Spark Streaming or Flink for real-time processing.
Handling of late or malformed events, checkpointing, fault tolerance.
Architecture Question 3: Data Warehouse vs. Data Lake
Scenario: Design a data platform that stores both structured transactional data and semi-structured logs, enabling analysts to perform queries.
Key Points to Discuss:
Differences between data lake (S3, ADLS) and data warehouse (Snowflake, BigQuery, Redshift).
ETL vs. ELT workflows.
Governance, schema evolution, and metadata management.
Architecture Question 4: High-Volume Ingestion
Scenario: You need to ingest millions of sensor readings per second from IoT devices and store them for historical analysis.
Key Points to Discuss:
Queue/buffer layer (Kafka, Kinesis).
Horizontal scaling of ingestion endpoints.
Time-series databases (InfluxDB, Timescale, or partitioned warehouse tables).
Architecture Question 5: Data Lakehouse Concept
Scenario: A company wants a unified platform for both SQL analytics and ML workloads on top of large volumes of raw data.
Key Points to Discuss:
Lakehouse design (e.g., Delta Lake, Apache Iceberg).
Transactional guarantees, ACID compliance.
Merging streaming and batch workloads with a single source of truth.
Architecture Question 6: Hybrid On-Prem & Cloud
Scenario: Migrate an existing on-prem Hadoop cluster partially to the cloud while retaining sensitive data in the local data centre.
Key Points to Discuss:
Data pipelines bridging on-prem and cloud (VPN, AWS Direct Connect, etc.).
Compliance and security constraints.
Orchestration tools that can manage hybrid workflows.
Architecture Question 7: Scalable NoSQL Setup
Scenario: You must handle high-write throughput with minimal latency, storing user session data in a distributed data store.
Key Points to Discuss:
NoSQL choices (Cassandra, DynamoDB, MongoDB).
Data modelling for speed (primary key design, partition keys).
Handling hot partitions or uneven traffic distribution.
Architecture Question 8: Machine Learning Feature Store
Scenario: Design a feature store to provide consistent, real-time features for ML models in both training and inference.
Key Points to Discuss:
Data ingestion from multiple systems.
Online vs. offline feature store.
Versioning and quality checks for features.
Architecture Question 9: CDC (Change Data Capture) Pipeline
Scenario: You need to replicate changes from an OLTP database to a data warehouse in near real time.
Key Points to Discuss:
Tools like Debezium, AWS DMS, or StreamSets for CDC.
Handling schema changes, primary key updates.
Ensuring exactly-once or at-least-once delivery semantics.
Architecture Question 10: Orchestration & Workflow Management
Scenario: Multiple ETL tasks need to run in sequence, with some parallel steps, and must produce outputs for subsequent processes.
Key Points to Discuss:
Use of orchestration frameworks (Airflow, Prefect, Luigi).
Defining DAGs (Directed Acyclic Graphs).
Error handling, retries, and alerting on failure.
Architecture Question 11: CI/CD for Data Pipelines
Scenario: Implement a continuous integration and continuous delivery process for Spark jobs or other data processing tasks.
Key Points to Discuss:
Version control and build pipelines (Jenkins, GitLab CI/CD).
Automated testing with sample datasets.
Canary or blue-green deployments in data contexts.
Architecture Question 12: Designing a Metadata Catalogue
Scenario: Analysts need a central place to discover what data sets exist and understand schemas, lineage, and ownership.
Key Points to Discuss:
Tools like Apache Atlas, Alation, or AWS Glue Data Catalog.
Automatic schema discovery vs. manual annotation.
Role-based access controls and integration with governance frameworks.
Architecture Question 13: Data Replication & High Availability
Scenario: A global e-commerce business requires data to be instantly available across multiple regions.
Key Points to Discuss:
Database replication strategies (multi-master, master-slave).
Conflict resolution in multi-master setups.
Latency vs. consistency trade-offs (CAP theorem).
Architecture Question 14: ETL vs. ELT in Cloud Environments
Scenario: You must integrate data from 10+ sources (API, DBs, CSV) into a central data store for analytics. Evaluate ETL vs. ELT.
Key Points to Discuss:
ETL and transformations on the fly vs. loading raw data (ELT) into a warehouse.
Tools like dbt for in-warehouse transformations.
Cost, performance, and data agility considerations.
Architecture Question 15: Observability & Monitoring
Scenario: Build a monitoring system that ensures data pipelines are up, transformations are correct, and data freshness is tracked.
Key Points to Discuss:
Logging and metric collection (Prometheus, Grafana, Splunk).
Data quality checks (Great Expectations, Deequ).
Alerting on SLA breaches or anomalies.
When discussing architecture solutions, always consider:
Scale: Will your design handle a 10x data growth?
Reliability: How will you ensure no data loss or duplication?
Security & Compliance: Encryption, role-based access, data governance.
Cost: Cloud usage can skyrocket if not carefully planned.
4. Tips for Conquering Data Engineering Job Interviews
Data engineering interviews can be challenging—combining coding, system design, and domain-specific knowledge. Here are key strategies to help you stand out:
Revisit CS Fundamentals
Brush up on algorithms, data structures, complexity analysis, and database design.
Many coding challenges test your problem-solving approach rather than advanced library usage.
Demonstrate Familiarity with Big Data Tools
Employers often use Spark, Hadoop, Kafka, Airflow, and other frameworks.
Show practical experience—mention how you overcame challenges like data skew in Spark or large messages in Kafka.
Know Your Cloud Platforms
Cloud experience is highly valued. Understand how to spin up and optimise data services on AWS, Azure, or GCP.
Familiarise yourself with services like AWS Glue, Azure Data Factory, or GCP Dataflow.
Talk Through Trade-Offs
When asked about architecture, emphasise pros and cons of different approaches.
For instance, discuss how a Kappa architecture might compare to a Lambda architecture for streaming.
Practise Whiteboard & Collaborative Coding
Interviews might involve writing SQL or Python on a whiteboard or in a shared online editor.
Clearly outline your logic, edge cases, and error handling. Think aloud so interviewers see your problem-solving process.
Highlight Data Quality & Governance
Data reliability is paramount. Mention the importance of data lineage, data validation, and observability.
Demonstrate knowledge of GDPR or other compliance requirements if relevant.
Show Team Collaboration
Data engineers often liaise with data scientists, analysts, and business leaders.
Employers value strong communication—explain how you’ve translated stakeholder needs into actionable data requirements.
Keep Up with Industry Trends
The data landscape evolves quickly (e.g., Data Lakehouse, real-time streaming frameworks).
Subscribe to blogs or attend meetups to stay informed about the latest developments.
Address Performance & Optimisation
Organisations spend heavily on compute. Demonstrate how you’d tune queries, partition data, or use caching to reduce costs and improve speed.
Cite examples where you improved pipeline efficiency or lowered resource usage.
Be Ready to Ask Questions
Show you’re engaged by inquiring about the organisation’s data stack, engineering culture, or future roadmaps.
This helps you assess if the company’s challenges align with your career goals.
A balanced mix of technical acumen, practical experience, and clear communication will significantly boost your interview performance.
5. Final Thoughts
Data engineering sits at the core of modern analytics and AI/ML initiatives, requiring deep knowledge of distributed computing, data pipelines, and cloud architectures. By studying the 30 real coding & system-design questions outlined here—covering everything from CSV parsing to scalable data lakes—you’ll sharpen the skills employers most desire.
Remember, interviews are a two-way conversation. While companies evaluate your expertise, you should also gauge whether their tech stack and culture match your ambitions. If you’re seeking the latest data engineering jobs in the UK, check out www.dataengineeringjobs.co.uk. The site features diverse opportunities across industries, enabling you to find the perfect fit for your skillset and career goals.
Approach your interview with confidence, a willingness to learn, and a solid grasp of both coding fundamentals and architectural best practices. Armed with this knowledge, you’ll be well on your way to impressing potential employers and securing a rewarding data engineering position.